C++ Classes and Objects
Class:- A class in C++ is the building block, that leads to Object-Oriented programming. It is a user-defined data type, which holds its own data members and member functions, which can be accessed and used by creating an instance of that class. A C++ class is like a blueprint for an object.
Class...
- Class is a collection of data member and member function in a single unit .
- Class is a keyword which is used for declare a class .
- The member of the class is private by default.
- Class is used for providing high security.
- Class is used in large program and it takes more memory space.
- Syntax :
{
Private :
...................
......…..........
Public :
...................
...................
};
* Object...
- Object is an instance of a class .
- We can pass the value directly through the object .
- Syntax : class_name object_name ;
Define C++ Objects
A class provides the blueprints for objects, so basically an object is created from a class. We declare objects of a class with exactly the same sort of declaration that we declare variables of basic types. Following statements declare two objects of class Box −
Box Box1; // Declare Box1 of type Box Box Box2; // Declare Box2 of type Box
Both of the objects Box1 and Box2 will have their own copy of data members.
The classes are the most important feature of C++ that leads to Object Oriented programming. Class is a user defined data type, which holds its own data members and member functions, which can be accessed and used by creating instance of that class.
The variables inside class definition are called as data members and the functions are called member functions.
For Example: Consider the Class of Cars. There may be many cars with different names and brand but all of them will share some common properties like all of them will have 4 wheels, Speed Limit, Mileage range etc. So here, Car is the class and wheels, speed limits, mileage are their properties.
- A Class is a user defined data-type which has data members and member functions.
- Data members are the data variables and member functions are the functions used to manipulate these variables and together these data members and member functions defines the properties and behavior of the objects in a Class.
- In the above example of class Car, the data member will be speed limit, mileage etc and member functions can be apply brakes, increase speed etc.
An Object is an instance of a Class. When a class is defined, no memory is allocated but when it is instantiated (i.e. an object is created) memory is allocated.
Concept of class with example
Example of class in easy way to understand In C++.
#include
#include
using namespace std;
// Class Declaration
class technical {
public:
string name;
int number;
};
int main() {
// Object created
technical obj;
cout << "Enter the Name :";
cin >> obj.name;
cout << "Enter the Number :";
cin >> obj.number;
cout << obj.name <<" : "<< obj.number << endl;
getch();
return 0;
}
Output
Enter the Name :point
Enter the Number :100
point
: 100
Defining Class and Declaring Objects
A class is defined in C++ using keyword class followed by the name of class. The body of class is defined inside the curly brackets and terminated by a semicolon at the end.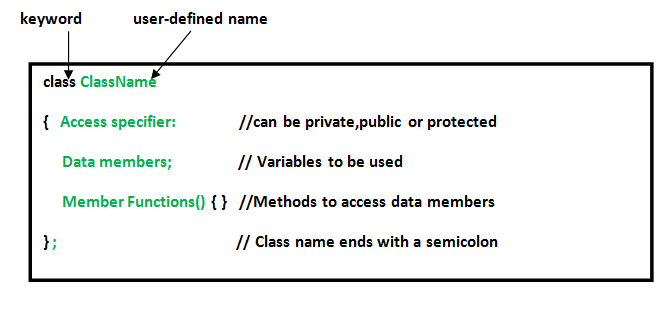
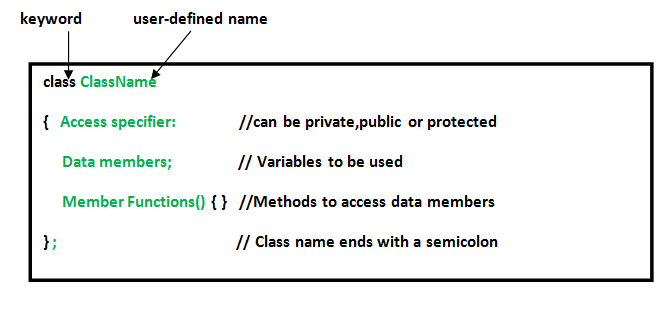
Declaring Objects: When a class is defined, only the specification for the object is defined; no memory or storage is allocated. To use the data and access functions defined in the class, you need to create objects.
Syntax:
ClassName ObjectName;
Accessing data members and member functions: The data members and member functions of class can be accessed using the dot(‘.’) operator with the object. For example if the name of object is obj and you want to access the member function with the name printName() then you will have to write obj.printName() .
Accessing Data Members
The public data members are also accessed in the same way given however the private data members are not allowed to be accessed directly by the object. Accessing a data member depends solely on the access control of that data member.
This access control is given by Access modifiers in C++. There are three access modifiers : public, private and protected.
This access control is given by Access modifiers in C++. There are three access modifiers : public, private and protected.
Output:
echnicalpointname is: Learning
Member Functions in Classes
There are 2 ways to define a member function:
- Inside class definition
- Outside class definition
To define a member function outside the class definition we have to use the scope resolution :: operator along with class name and function name.
Output:
Geekname is: xyz Geek id is: 15
Note that all the member functions defined inside the class definition are by default inline, but you can also make any non-class function inline by using keyword inline with them. Inline functions are actual functions, which are copied everywhere during compilation, like pre-processor macro, so the overhead of function calling is reduced.
Note: Declaring a friend function is a way to give private access to a non-member function.
c++ - Returning object from function
A function can also return objects either by value or by reference. When an object is returned by value from a function, a temporary object is created within the function, which holds the return value. This value is further assigned to another object in the calling function
The syntax for defining a function that returns an object by value is
class_name function_name (parameter_list)
{
// body of the function
}
To understand the concept of returning an object by value from a function, consider this example.
Example of Passing object & Returning object
#include#include class Rectangle { int L,B; Rectangle(int l,int b) { L = l; B = b; } Rectangle() { L = 0; B = 0; } Rectangle Sum(Rectangle Rec) //function 1 { Rectangle temp; temp.L = L + Rec.L; //Statement 1 temp.B = B + Rec.B; //Statement 2 return temp; } void Display() { cout<<"\nLength : "< //Statement 3
In statement 3, object R1 is calling member function Sum and passing object R2 as argument. Function 1 is receiving values of R2 in Rec. In statement 1 L is length of calling object R1 and Rec.L is length of object R2.
Both statement 1 and 2 is adding the length and breadth of Rectangle 1 and 2 respectively and assigning the result to object temp.
Statement 3 is receiving the values of object temp in object R3.
Structure vs class in C++
In C++, a structure is the same as a class except for a few differences. The most important of them is security. A Structure is not secure and cannot hide its implementation details from the end user while a class is secure and can hide its programming and designing details. Following are the points that expound on this difference:
1) Members of a class are private by default and members of a struct are public by default.
For example program 1 fails in compilation and program 2 works fine.
For example program 1 fails in compilation and program 2 works fine.
Example for Array of object
#includeEnter details of 1 Employee#include class Employee { int Id; char Name[25]; int Age; long Salary; public: void GetData() //Statement 1 : Defining GetData() { cout<<"\n\tEnter Employee Id : "; cin>>Id; cout<<"\n\tEnter Employee Name : "; cin>>Name; cout<<"\n\tEnter Employee Age : "; cin>>Age; cout<<"\n\tEnter Employee Salary : "; cin>>Salary; } void PutData() //Statement 2 : Defining PutData() { cout<<"\n"< Output :
Enter Employee Id : 101
Enter Employee Name : Mohsin
Enter Employee Age : 20
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Naim
Enter Employee Age : 20
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Mirza Ali
Enter Employee Age : 21
Enter Employee Salary : 47000
Details of Employees
101 Mohsin 20 45000 1
02 Naim 20 51000
103 Mirza Ali 28 47000
No comments:
Post a Comment